diskpart
list disk
select disk <the disk number>
clean
Check when Active Directory password expires
net user /domain <username> | findstr /i expires
Share a new Angular module with multiple standalone components
// material-components.module.ts
const modules = [
CommonModule,
MatAutocompleteModule,
MatButtonModule,
MatCardModule,
MatDialogModule,
MatFormFieldModule,
MatInputModule,
MatListModule,
MatSortModule,
MatTableModule
];
@NgModule({
imports: modules,
exports: modules
})
export class MaterialComponentsModule { }
// home.component.ts
@Component({
selector: 'app-home',
standalone: true,
imports: [MaterialComponentsModule],
templateUrl: './home.component.html',
styleUrl: './home.component.scss'
})
export class HomeComponent {
Home Dashboard V2
I’ve updated the touchscreen dashboard to have larger buttons, and I’ve completed the Hydrawise Sprinkler integration. Everything is fully functional now!
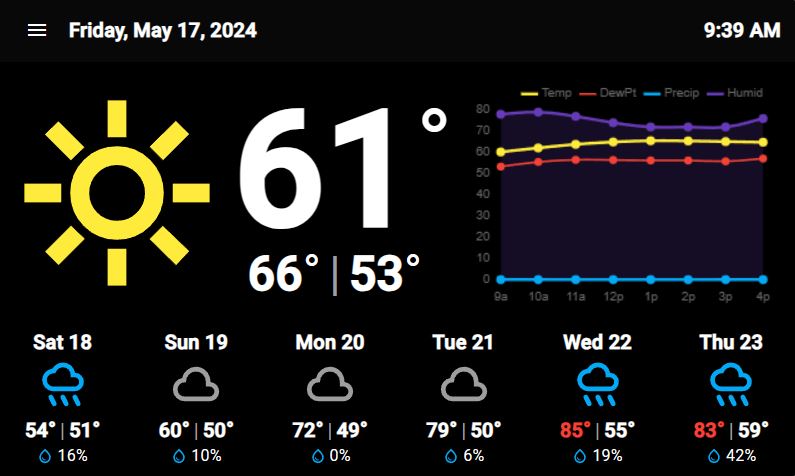
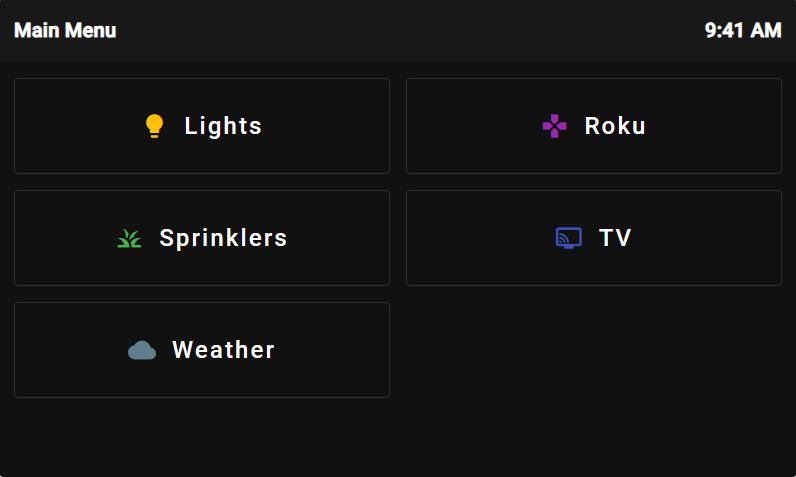
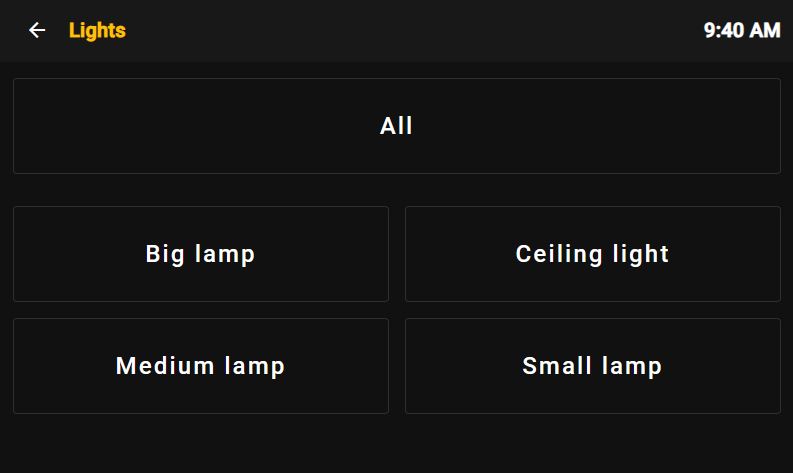
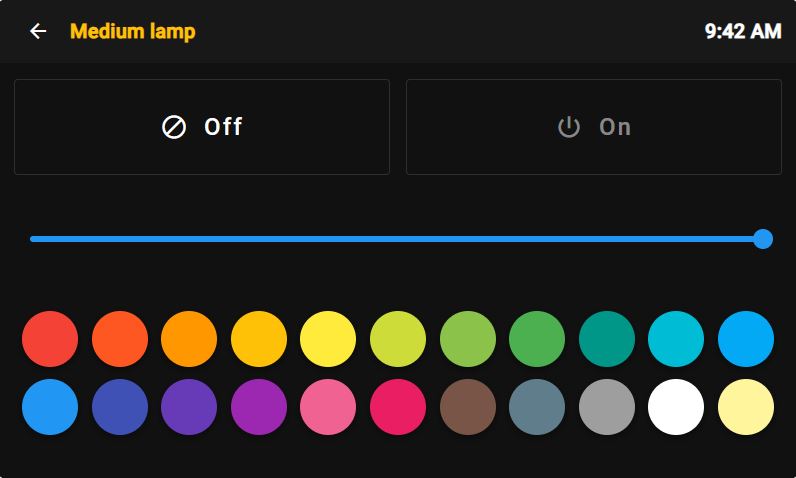
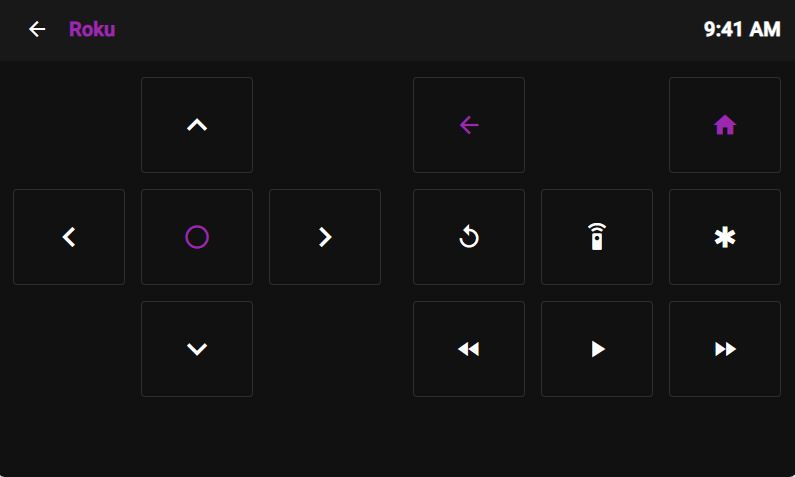
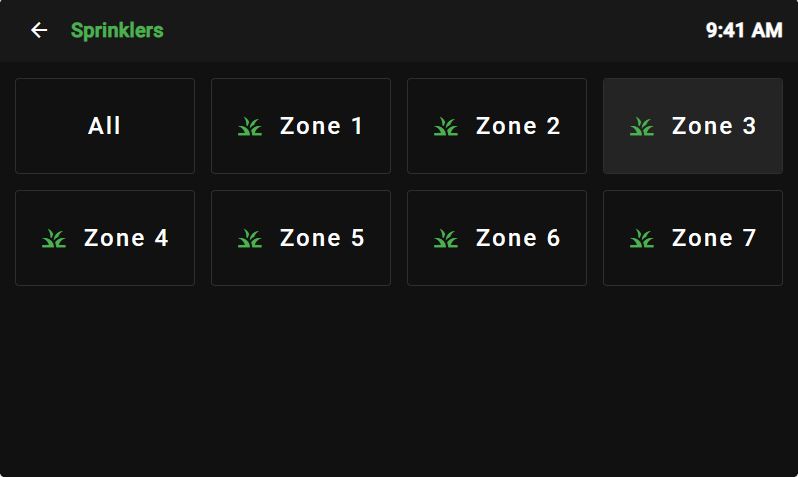
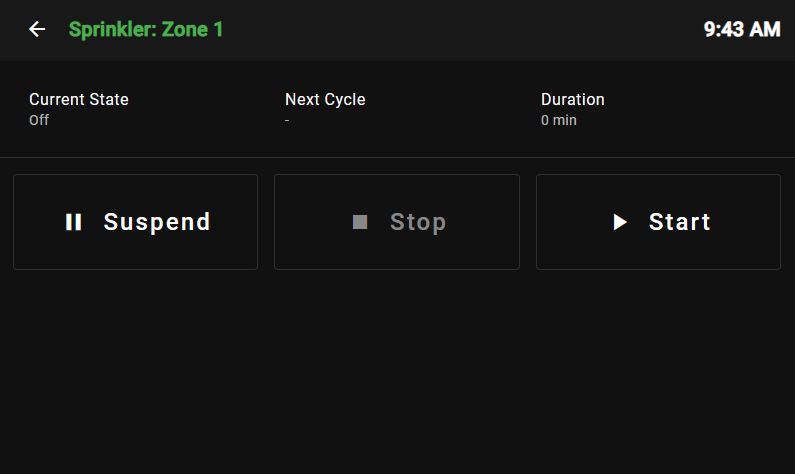
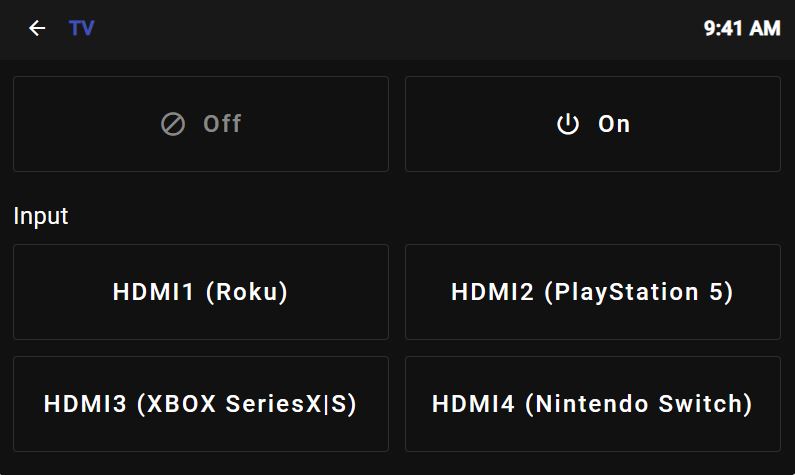
Home Dashboard
This web application is hosted on my local IIS server and is displayed on a Raspberry Pi w/ touchscreen. It’s 100% custom, using Angular Material with a dotnet backend. It communicates with several IoT APIs, including:
- Philips Hue
- Samsung SmartThings
- Hydrawise
- OpenMeteo
- Roku
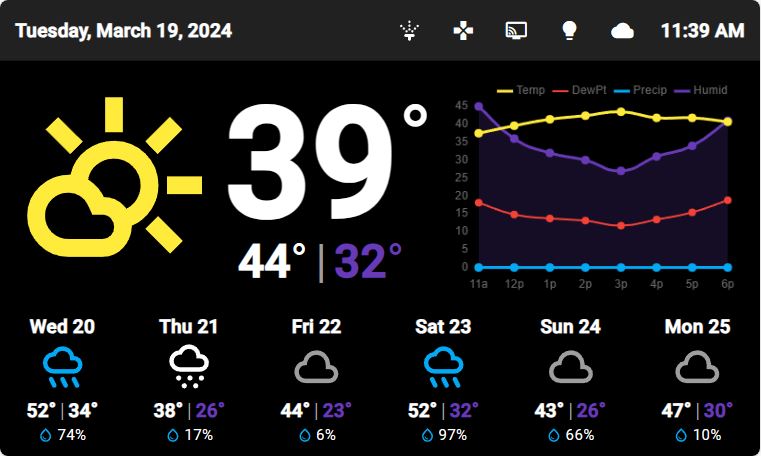
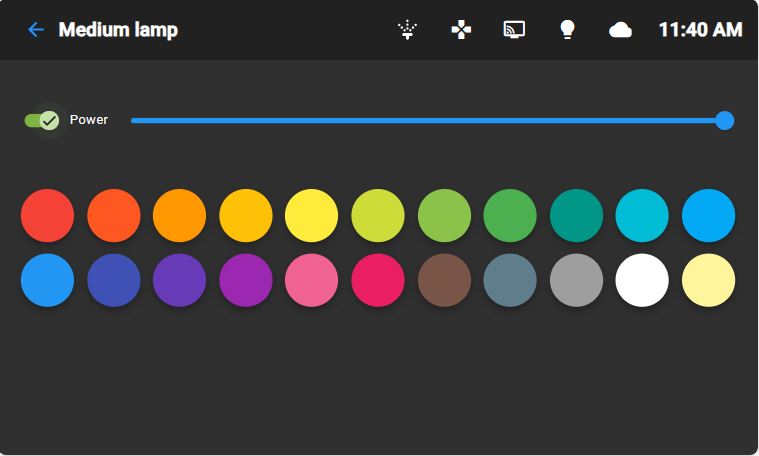
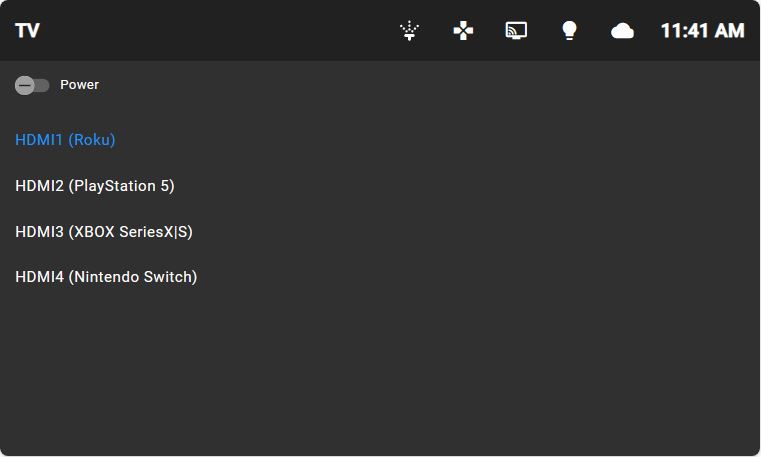
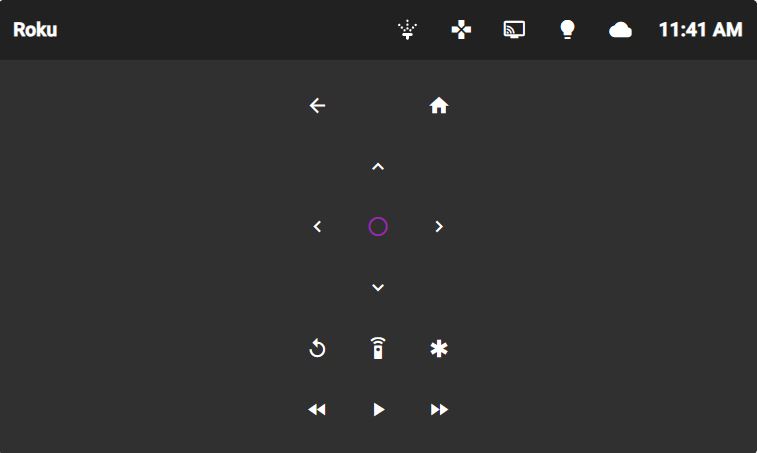
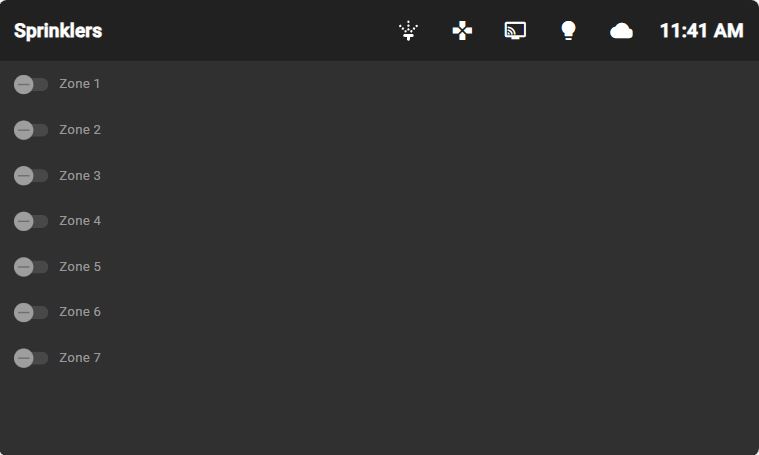
Repeat an observable in Angular (RXJS)
interval(1000).subscribe(() => {
this._getLatest().subscribe(latest => {
//...
});
});
Set static IP in linux
determine the name of your network interface
ip a
make a backup of the netplan configuration
sudo cp /etc/netplan/01-network-manager-all.yaml /etc/netplan/01-network-manager-all.yaml.backup
edit the config file
sudo vim.tiny /etc/netplan/01-network-manager-all.yaml
make the following changes, replacing “interface_name” with the intended interface
network:
version: 2
renderer: networkd
ethernets:
interface_name:
addresses:
- 192.168.1.101/24
nameservers:
addresses: [8.8.8.8]
routes:
- to: default
via: 192.168.1.1
try the new configuration
sudo netplan try
If all is good, press ENTER
Lego Deals
This application displays the current prices of Lego sets at major retailers. Every hour, a scheduled task scrapes 29 retailer websites for their discounted Lego sets, and those prices are saved to a database. I started collecting the hourly price history in December 2022 and it continues to run today. I’m able to set alerts via Telegram when the price of a particular set drops below a given threshold. The application also keeps track of which sets I already own, which ones I am interested in, and which ones I never want to see. I can switch to a view that only shows sets I care about.
Lego set details, like number of pieces, are pulled from Rebrickable’s daily dataset. Once per day, a scheduled task downloads the latest Rebrickable dataset and adds the new entries to my database. MSRP is scraped from the Lego website and the BestBuy API. BestBuy just happens to have the best API of all the retailers. And it’s FREE!
Another page in the application displays Ebay buy-it-now, free shipping listings. Due to inconsistency with Ebay data, these prices are not tracked in my database. Ebay deals are displayed on a totally separate page from the retailer listings; however, the Ebay page can be filtered by the same criteria.
The backend, dotnet API and Angular application are hosted on a local IIS server. Several scheduled tasks, like MSRP updates, Rebrickable datasets, alerts, etc. run on the same server. The MySQL database is hosted on Bluehost.
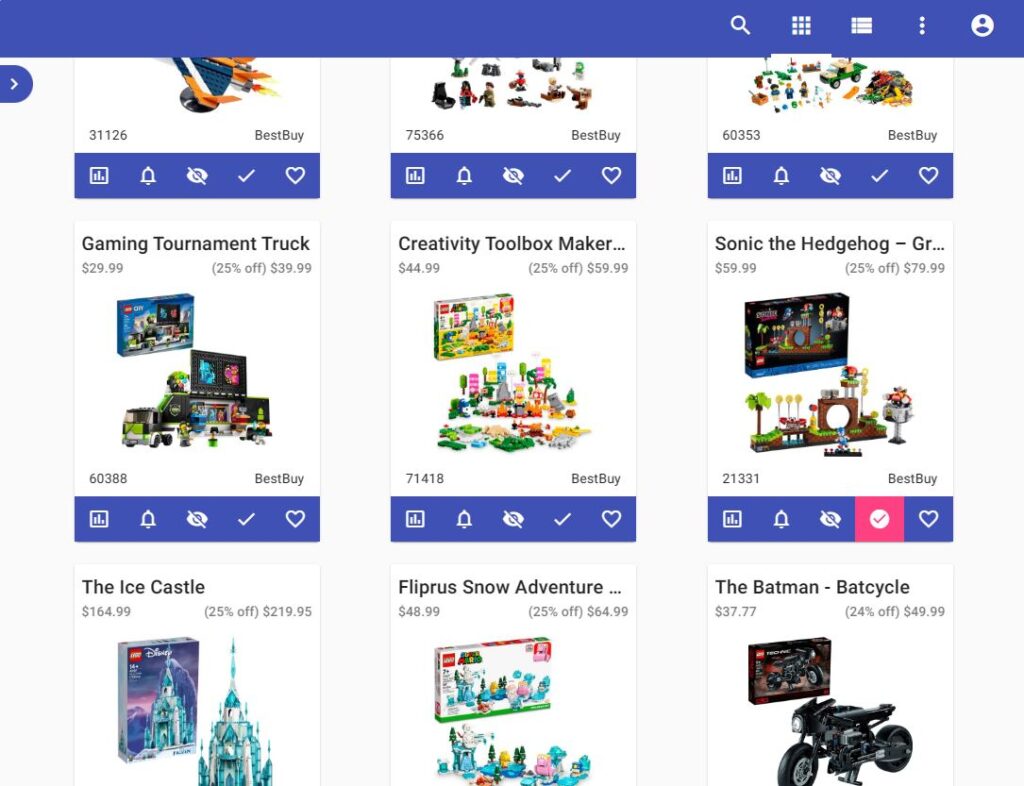
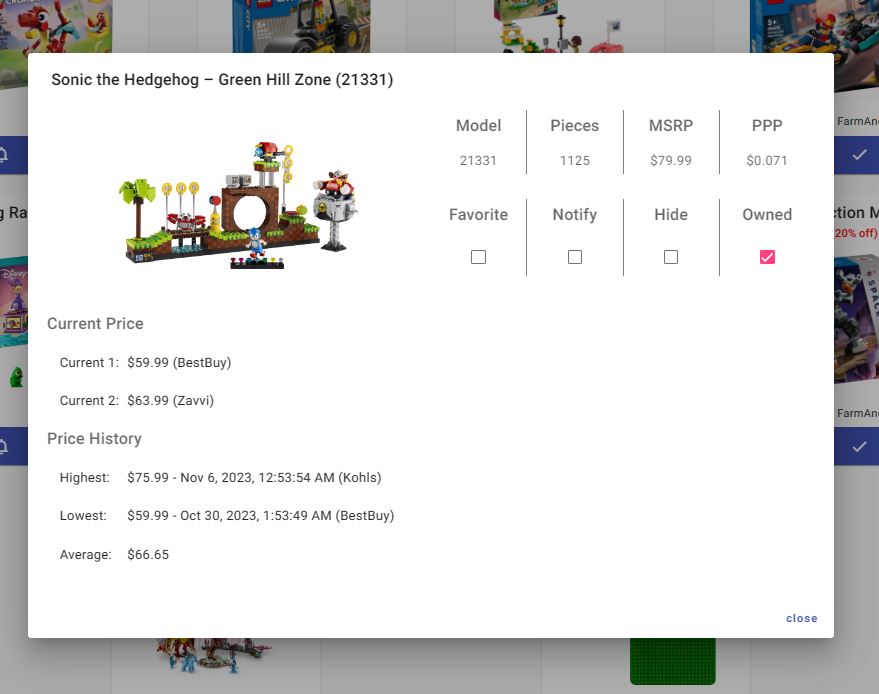
Insert into a MsSQL table that only has auto-generated columns
INSERT INTO table_name DEFAULT VALUES
New angular app is missing environment.ts and environment.prod.ts
The ng new
command no longer automatically includes environments. You must run the following:
ng g environments
Disable the Windows “Shake” feature
Shaking the mouse while dragging another window’s title bar minimizes all other Windows. The UI to disable this “feature” is no longer obvious and I was able to disable it by making the following registry change. Note: This worked even on a gpo machine since it only modified CURRENT_USER.
registry location
HKEY_CURRENT_USER\SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\Advanced
Add the following DWORD key with value 1
DisallowShaking
Angular pipe to replace characters in a string
Generate a new pipe using the Angular CLI
ng g pipe pipes/replace
Code inside the new pipe (pipes/replace.pipe.ts)
...
transform(input: string, from: string, to: string): string {
const regEx = new RegExp(from, 'g');
return input.replace(regEx, to);
}
Usage
// page.component.html
// replaces underscores with spaces
...
<span>{{ myString | replace:'_':' '}}</span>
Display enums as strings in Swagger/Swashbuckle (.net6)
// Program.cs
builder.Services.AddControllers().AddJsonOptions(opt =>
{
opt.JsonSerializerOptions.Converters.Add(new JsonStringEnumConverter());
});
Mock a non-singleton Angular service in a component unit test
describe('MyComponent', () => {
let mockService: jasmine.SpyObj<MyService>;
beforeEach(async () => {
mockService = jasmine.createSpyObj<MyService>(['download']);
await TestBed.configureTestingModule({
...
}).overrideComponent(MyComponent, {
set: {
providers: [
{ provide: MyService, useValue: mockService }]
}
}).compileComponents();
beforeEach(() => {
...
});
it('should create', () => {
...
});
});